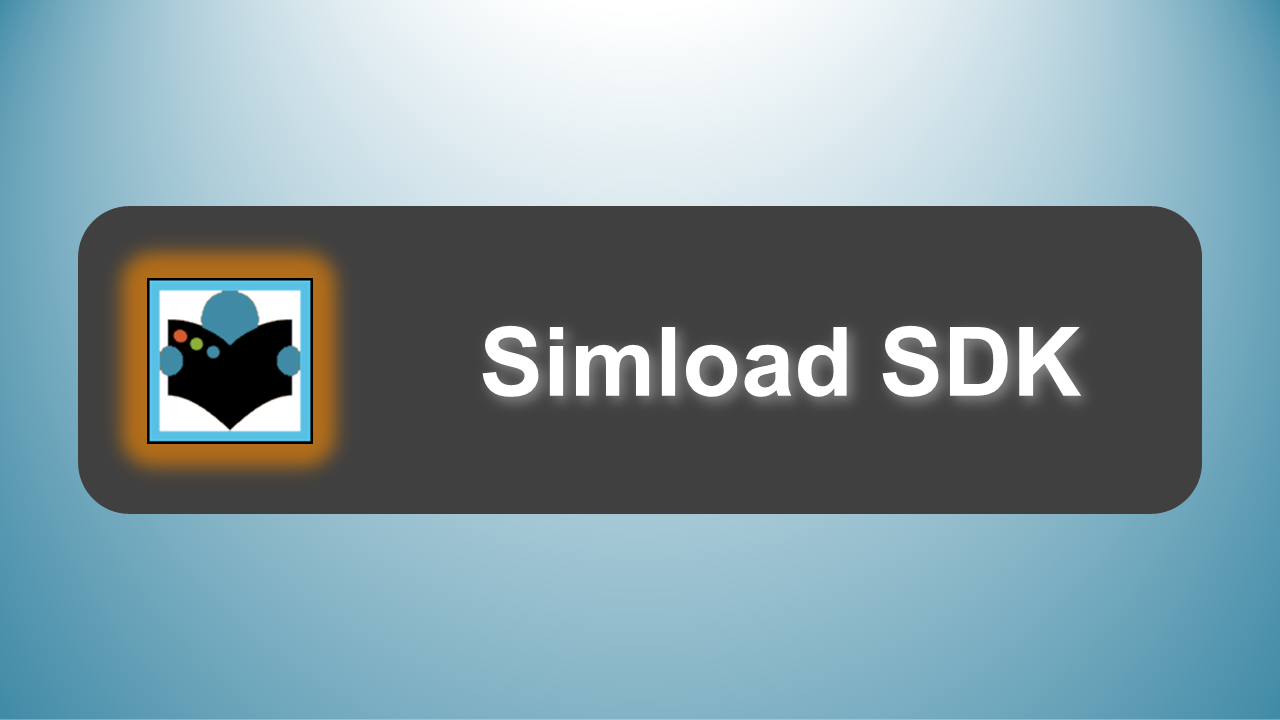
[EUC Score Toolset Documentation Start Page]
The Install-SDK package includes libraries, tools and sample code needed to build your own Simloads.
Components required for using the Simload SDK.
Simload code design principles and conventions.
Simload examples with references to all required source files.
The Simload Install-SDK package includes sample code, libraries, icons and some extra tools. It requires the Install-EUCScore package to be installed on the target machine. The preferred development environment for EUC Score Simloads is Visual Studio Code plus extensions.
Visual Studio Code is a lightweight source code editor which is available for Windows. It has a rich ecosystem of extensions for other languages and runtimes, such as PowerShell, AutoIt and Inno Setup.
An installed AutoIt v3 is a prerequisite for the Simload SDK. AutoIt is a freeware BASIC-like scripting language designed for automating the Windows GUI and general scripting. It uses a combination of simulated keystrokes, mouse movement and window control manipulation. AutoIt is very small and only consumes minimal system resources. Scripts can be compiled into self-contained executables that include all runtime components and run on all versions of Windows out-of-the-box. In addition to the runtime components, the AutoIt install package includes the SciTE editor that provides syntax highlighting.
The AutoIt extension for Visual Studio Code replaces the SciTE editor, but still requires the installed AutoIt development environment, including the compiler. In Visual Studio Code, search for the AutoIt extension by Damien and install it. By default, the extension is set up for the default installation of AutoIt on a 64-bit system. For alternate setups, you can change the configuration by navigating to File-> Preferences-> Settings.
Another Simload SDK prerequisite is an installed Inno Setup. It is a free installer for Windows programs by Jordan Russell and Martijn Laan, allowing the creation of a single EXE for easy online distribution. An important feature is the installation of 64-bit applications on the 64-bit editions of Windows. Both administrative and non administrative installations are supported as well as the creation of registry and INI entries. For details, see Inno Setup Help
The Inno Setup extension for Visual Studio Code provided by idleberg is a language syntax, snippets and build system for Inno Setup. Before you can build an Inno Setup package, make sure the compiler ISCC is in your PATH environmental variable. Alternatively, you can specify the path to ISCC in your user settings. To trigger a build, select "Inno Setup: Save & Compile Script" from the command-palette or use the default keyboard shortcut SHIFT+ALT+B.
IMPORTANT NOTE: The development of custom Simloads does not rely on Visual Studio Code, AutoIt and Inno Setup! For the Simload code, any programming language is suitable that can be compiled into a Windows executable and that allows to manipulate Windows programs, text files and registry entries. Creating an installation package with Inno Setup is also optional as a copy and paste deployment of Simloads is supported.
The code behind each EUC Simload is separated into multiple modules. A type 1 Simload located in Simloads\<GroupName> has a main code file named SL1-<SimloadName>.au3. An include or library file located in the same folder — named Lib-<SimloadName>.au3 — contains Simload-specific functions. The purpose of these functions is to provide a mechanism to reuse code for Simload-specific user actions in type 2 Simloads (= Personas).
Another mandatory module located in the same folder is the SL1-<SimloadName>.ini file. This side-by-side .ini file can be used to overwrite the hard-coded Simload default settings as defined in the main code file. It also acts as a fallback in case general Simload settings and folder locations are not stored in the registry.
The general include or library file LibSimloads.au3 located in the Libs folder contains a spectrum of common functions: Main Functions, Temp Object Functions, Process Functions and App Functions. Many of the functions write to or read from a Temporary Simload Object. The code in LibSimloads.au3 follows the design guidelines of AutoIt User Defined Functions (UDFs).
Simload-Specific Functions in Simloads\<GroupName>\Lib-<SimloadName>.au3
Main Functions in Libs\LibSimLoads.au3
Temporary Object Functions in Libs\LibSimLoads.au3
Process Functions in Libs\LibSimLoads.au3
Windows App Functions in Libs\LibSimLoads.au3
The SDK install package adds the following folders and files:
Folder | File Name | Description |
---|---|---|
Libs | ||
LibSimLoads.au3 | Main Simload user-defined function library. | |
Simloads\ZL1-Samples | ||
Compile-ZL1-Samples.au3 | Running this AutoIt file with F5 compiles the Simloads in this folder. | |
ZL1-Empty.au3 | AutoIt sample source code with an empty Simload object that can be used as a template. Many lines with comments were added with the purpose of making the source code easier to understand. | |
ZL1-Empty.ini | Side-by-side .ini file for the ZL1-Empty Simload with empty Simload object. The section name [ZL1-Empty] must reflect the code file name without extension. The same is true for the keyname in the [Simloads] section and the path to the Simload executable in the value. | |
Lib-Empty.au3 | Simload-specific functions: _SimLoad_EmptyInit, _SimLoad_EmptyRunAction, _SimLoad_EmptyTerminate, _SimLoad_EmptyStartAction and _SimLoad_EmptyStopAction. | |
ZL1-Empty.exe | The executable of the ZL1-Empty Simload after it was compiled using Compile-ZL1-Samples.au3. | |
ZL1-App.au3 | AutoIt sample source code with an empty Simload object that relies on application configuration details in the side-by-side .ini file. While the ZL1-App Simload is running, a dialog box with temporary object values is show in the bottom right corner of the screen. | |
ZL1-App.ini | Side-by-side .ini file for the ZL1-App Simload with all required Simload object values. The section name [ZL1-App], the keyname in the [Simloads] section and the path to the Simload executable in the value must be set properly. | |
Lib-App.au3 | Simload-specific functions: _SimLoad_AppInit, _SimLoad_AppRunAction, _SimLoad_AppTerminate, _SimLoad_AppStartAction and _SimLoad_AppStopAction. The additional function _SimLoad_ShowForm displays a dialog box with temporary object values in the bottom right corner of the screen. | |
ZL1-App.exe | The executable of the ZL1-App Simload after it was compiled using Compile-ZL1-Samples.au3. | |
ZL1-NotepadTest.au3 | AutoIt sample source code with a Simload object that is preconfigured to open Notepad. The side-by-side ini file can be used to overwrite object values. | |
ZL1-NotepadTest.ini | Side-by-side .ini file for the ZL1-NotepadTest Simload with optional Simload object values. The section name [ZL1-NotepadTest], the keyname in the [Simloads] section and the path to the Simload executable in the value must be set properly. | |
Lib-NotepadTest.au3 | Simload-specific functions: _SimLoad_AppInit, _SimLoad_AppRunAction, _SimLoad_AppTerminate, _SimLoad_AppStartAction and _SimLoad_AppStopAction. | |
ZL1-NotepadTest.exe | The executable of the ZL1-NotepadTest Simload after it was compiled using Compile-ZL1-Samples.au3. | |
ZL1-MSEdgeTest.au3 | AutoIt sample source code with a Simload object that is preconfigured to open a web page in Microsoft Edge. The run action scrolls up and down every second. The side-by-side ini file can be used to overwrite object values. | |
ZL1-MSEdgeTest.ini | Side-by-side .ini file for the ZL1-MSEdgeTest Simload with optional Simload object values. The section name [ZL1-NotepadTest], the keyname in the [Simloads] section and the path to the Simload executable in the value must be set properly. | |
Lib-MSEdgeTest.au3 | Simload-specific functions: _SimLoad_AppInit, _SimLoad_AppRunAction, _SimLoad_AppTerminate, _SimLoad_AppStartAction and _SimLoad_AppStopAction. | |
ZL1-MSEdgeTest.exe | The executable of the ZL1-MSEdgeTest Simload after it was compiled using Compile-ZL1-Samples.au3. | |
Tools | ||
WinInfo.exe | Table showing process ID, window handle, file name, window title, position, size and state of all enabled and visible windows. | |
WinInfoPlus.exe | Table showing process ID, window handle, file name, window title, position, size, state, username and domain of all enabled and visible windows. A file with the results is stored in c:\Users\Public\Documents\. | |
ProcInfo.exe | Table showing process name, process ID and file name of all processes running on the system. A file with the results is stored in c:\Users\Public\Documents\. | |
WtsProcInfo.ex | Table showing process name, process ID, file name, session ID and user info of all processes running on the system, using the Terminal Server API. A file with the results is stored in c:\Users\Public\Documents\. | |
TempObjectInfo.exe | Dialog box allowing to select a temporary object and show all values. | |
Icons | ||
EUCScore_AppTimeLarge.ico | Icon used for type 1 Simloads. | |
EUCScore_AppSet.ico | Icon used for type 2 Simloads (Personas). |
The Inno Setup file Install-ZL1-Samples.iss in the EUC Score root folder contains the source code required to build the SDK install package. The target folder is \Installers.